I've been researching the holiday theme craze of music light shows using vixen and Arduino for months now. I have already done a lot of testing of the lights using the basic code and setup example offered for the web and on these forums. I have made progress in some areas and little in others, I am quite experienced with wiring electronics but im not so great with the coding and DATA transmission options.
Here is the Goal: I will be using Vixen lights, I would like to use the newer teensy 4.0 controller; 1 of them for a pixel tree(With the octows2811 adapter). The other teensy for a lengthy strand of ws2811 pixels from the corner of my garage to the opposite corner of the house. I will also most likely use Arduino Nanos for smaller runs of ws2811s around doorframes, windows, ect. IN ADDITION TO the use of ws2811 strips, I'd like at least one solid-state relay board to use with traditional plug-in lighting switched by the relays.
Having known the goal I have, I know there are many routes to take as far as data transmission and coding goes. BECAUSE I'm not sure I can trust my slow internet connection where I live, I am unsure if using the esp8266 wifi transmit/receive modules will suit me the best for signals from a computer in the house to controllers to lights. I am considering the use of RS-485 modules, to transmit the data signals physically over the wire to controllers from pc.
I've come as far as testing 4 strands of ws2811s across 4 pins on Arduino mega. This is as far as I can go without implementing rs-485, or the possible esp8266 modules. Okay.... I'll stop talking, you probably want to know the questions...
Question1: I am running vixen over usb to Arduino Mega, I finally found some code that made sense for what I want to accomplish, which is to run multiple addressable strands of ws2811 strands over the same number of pins on the mega(for testing anyway; later to be used on teensy). EACH PIN addressable in vixen. I have somewhat achieved this. When testing the strands, I keep getting funky situations. when I manually test an element in vixen, 1 strand will light up correctly, but then a number of bulbs on a different strand will also light up, starting from the beginning of the strip and going to no more than 9 bulbs on average down that strand, sometimes the color of these bulbs are correct and sometimes not. A few strands have this issue, and the others do now. I have included 330R resisters into the data lines from the mega pins. I do not believe that I am getting data corruption from power lines. I need help figuring out if this issue is from the wiring, the code, or a combination of setup in vixen and code.
Here is a simple diagram of the setup.
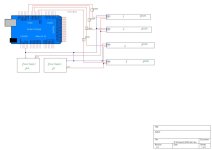
I'm using 2 power supplies, why? Cause I can I suppose, One is rated for 25 Amps, the other, only 3 Amps.
here is the code:
Question 2: Since I am likely going to be using RS-485, is there anything i need to change with the code above to incorporate the data transmission? Or is it as simple as wiring up the receiving and transmitting ends of the modules themselves? Or does anyone know how I can set up the esp8266 for testing with this code when I get them in the mail?
Question 3: Knowing the goal listed at the top of this post, I want to use 2 teensy, probably 4-6 Nanos(for windows) and a mega for the solid-state relays, If I am using rs-485... that's a lot of serial ports, I will obviously need to get a USB hub extender. But at that expense, could I expect slower display speeds because of the large mess of data my computer will be spitting out from vixen? Would the esp8266 be better after all in that sense?
Here is the Goal: I will be using Vixen lights, I would like to use the newer teensy 4.0 controller; 1 of them for a pixel tree(With the octows2811 adapter). The other teensy for a lengthy strand of ws2811 pixels from the corner of my garage to the opposite corner of the house. I will also most likely use Arduino Nanos for smaller runs of ws2811s around doorframes, windows, ect. IN ADDITION TO the use of ws2811 strips, I'd like at least one solid-state relay board to use with traditional plug-in lighting switched by the relays.
Having known the goal I have, I know there are many routes to take as far as data transmission and coding goes. BECAUSE I'm not sure I can trust my slow internet connection where I live, I am unsure if using the esp8266 wifi transmit/receive modules will suit me the best for signals from a computer in the house to controllers to lights. I am considering the use of RS-485 modules, to transmit the data signals physically over the wire to controllers from pc.
I've come as far as testing 4 strands of ws2811s across 4 pins on Arduino mega. This is as far as I can go without implementing rs-485, or the possible esp8266 modules. Okay.... I'll stop talking, you probably want to know the questions...
Question1: I am running vixen over usb to Arduino Mega, I finally found some code that made sense for what I want to accomplish, which is to run multiple addressable strands of ws2811 strands over the same number of pins on the mega(for testing anyway; later to be used on teensy). EACH PIN addressable in vixen. I have somewhat achieved this. When testing the strands, I keep getting funky situations. when I manually test an element in vixen, 1 strand will light up correctly, but then a number of bulbs on a different strand will also light up, starting from the beginning of the strip and going to no more than 9 bulbs on average down that strand, sometimes the color of these bulbs are correct and sometimes not. A few strands have this issue, and the others do now. I have included 330R resisters into the data lines from the mega pins. I do not believe that I am getting data corruption from power lines. I need help figuring out if this issue is from the wiring, the code, or a combination of setup in vixen and code.
Here is a simple diagram of the setup.
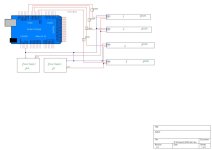
I'm using 2 power supplies, why? Cause I can I suppose, One is rated for 25 Amps, the other, only 3 Amps.
here is the code:
/*
Vixen Lights 3.x - Arduino Generic Serial for Addressable Pixels
Using this code is pretty straight forward, simply hookup your one wire (WS2811 or WS2812) data line to pin 6 of your Arduino
and upload this code. Make sure you have properly installed the FastLED library from http://fastled.io
Once you are done, simply
power your Pixel strips from an external power supply. Next configure a Generic Serial Controller inside of Vixen Lights 3.x and
add 3 x pixels for the number of channels. Configure the Generic Serial Controller to use 115200, 8, none, and 1. Then create
your element and add "Multiple Items (1 x number of pixels). Finally select your pixel elements and set them as RGB pixels before
patching them to the controler outputs. You should now be ready to begin testing.
For a complete tutorial check out blog.huntgang.com
Created November 8th, 2014
By Richard Sloan - www.themindfactory.com
And David Hunt - blog.huntgang.com
Version 1.4
*/
// You must download and install the library from http://fastled.io/
#include <FastLED.h>
// Sets the maximum number of LEDs that this code will handle to avoid running out of memory
#define NUM_LEDS 600 //Total leds of strip A+B+C+D
#define NUM_LEDS_A 150 //Strip A
#define NUM_LEDS_B 150 //Strip B
#define NUM_LEDS_C 150 //Strip C
#define NUM_LEDS_D 150 //Strip D
// Sets the pin which is used to connect to the LED pixel strip
#define DATA_PIN_A 2
#define DATA_PIN_B 4
#define DATA_PIN_C 6
#define DATA_PIN_D 8
CRGB ledsA[NUM_LEDS_A];
CRGB ledsB[NUM_LEDS_B];
CRGB ledsC[NUM_LEDS_C];
CRGB ledsD[NUM_LEDS_D];
void setup() {
// Define the speed of the serial port
Serial.begin(115200);
}
void loop() {
// Set some counter / temporary storage variables
/// int cnt;
int cnt_A;
int cnt_B;
int cnt_C;
int cnt_D;
unsigned int num_leds; ///Total leds named in the HEADER(vixen software)
unsigned int d1, d2, d3;
int NLA;
int NLB;
int NLC;
int NLD;
// Begin an endless loop to receive and process serial data
for(;
{
// Set a counter to 0. This couter keeps track of the pixel colors received.
/// cnt = 0;
cnt_A = 0;
cnt_B = 0;
cnt_C = 0;
cnt_D = 0;
NLA=NUM_LEDS_A;
NLB=NUM_LEDS_B;
NLC=NUM_LEDS_C;
NLD=NUM_LEDS_D;
//Begin waiting for the header to be received on the serial bus
//1st character
while(!Serial.available());
if(Serial.read() != '>') {
continue;
}
//second character
while(!Serial.available());
if(Serial.read() != '>') {
continue;
}
//get the first digit from the serial bus for the number of pixels to be used
while(!Serial.available());
d1 = Serial.read();
//get the second digit from the serial bus for the number of pixels to be used
while(!Serial.available());
d2 = Serial.read();
//get the third digit from the serial bus for the number of pixels to be used
while(!Serial.available());
d3 = Serial.read();
//get the end of the header
while(!Serial.available());
if(Serial.read() != '<') {
continue;
}
while(!Serial.available());
if(Serial.read() != '<') {
continue;
}
// calculate the number of pixels based on the characters provided in the header digits
num_leds = (d1-'0')*100+(d2-'0')*10+(d3-'0');
// ensure the number of pixels does not exceed the number allowed
if(num_leds > NUM_LEDS) {
continue;
}
// Let the FastLED library know how many pixels we will be addressing
FastLED.addLeds<WS2811, DATA_PIN_A, GRB>(ledsA, NUM_LEDS_A);
FastLED.addLeds<WS2811, DATA_PIN_B, RGB>(ledsB, NUM_LEDS_B);
FastLED.addLeds<WS2811, DATA_PIN_C, BGR>(ledsC, NUM_LEDS_C);
FastLED.addLeds<WS2811, DATA_PIN_D, RGB>(ledsD, NUM_LEDS_D);
// Loop through each of the pixels and read the values for each color
do {
while(!Serial.available());
ledsA[cnt_A].r = Serial.read();
while(!Serial.available());
ledsA[cnt_A].g = Serial.read();
while(!Serial.available());
ledsA[cnt_A++].b = Serial.read();
--num_leds;
}
while(--NLA);
/////// Loop through each of the pixels and read the values for each color
do {
while(!Serial.available());
ledsB[cnt_B].r = Serial.read();
while(!Serial.available());
ledsB[cnt_B].g = Serial.read();
while(!Serial.available());
ledsB[cnt_B++].b = Serial.read();
--num_leds;
}
while(--NLB);
////// Loop through each of the pixels and read the values for each color
do {
while(!Serial.available());
ledsC[cnt_C].r = Serial.read();
while(!Serial.available());
ledsC[cnt_C].g = Serial.read();
while(!Serial.available());
ledsC[cnt_C++].b = Serial.read();
--num_leds;
}
while(--NLC);
// Loop through each of the pixels and read the values for each color
do {
while(!Serial.available());
ledsD[cnt_D].r = Serial.read();
while(!Serial.available());
ledsD[cnt_D].g = Serial.read();
while(!Serial.available());
ledsD[cnt_D++].b = Serial.read();
--num_leds;
}
while(--NLD);
// Tell the FastLED Library it is time to update the strip of pixels
FastLED.show();
// WOO HOO... We are all done and are ready to start over again!
}
}
Vixen Lights 3.x - Arduino Generic Serial for Addressable Pixels
Using this code is pretty straight forward, simply hookup your one wire (WS2811 or WS2812) data line to pin 6 of your Arduino
and upload this code. Make sure you have properly installed the FastLED library from http://fastled.io
Once you are done, simply
power your Pixel strips from an external power supply. Next configure a Generic Serial Controller inside of Vixen Lights 3.x and
add 3 x pixels for the number of channels. Configure the Generic Serial Controller to use 115200, 8, none, and 1. Then create
your element and add "Multiple Items (1 x number of pixels). Finally select your pixel elements and set them as RGB pixels before
patching them to the controler outputs. You should now be ready to begin testing.
For a complete tutorial check out blog.huntgang.com
Created November 8th, 2014
By Richard Sloan - www.themindfactory.com
And David Hunt - blog.huntgang.com
Version 1.4
*/
// You must download and install the library from http://fastled.io/
#include <FastLED.h>
// Sets the maximum number of LEDs that this code will handle to avoid running out of memory
#define NUM_LEDS 600 //Total leds of strip A+B+C+D
#define NUM_LEDS_A 150 //Strip A
#define NUM_LEDS_B 150 //Strip B
#define NUM_LEDS_C 150 //Strip C
#define NUM_LEDS_D 150 //Strip D
// Sets the pin which is used to connect to the LED pixel strip
#define DATA_PIN_A 2
#define DATA_PIN_B 4
#define DATA_PIN_C 6
#define DATA_PIN_D 8
CRGB ledsA[NUM_LEDS_A];
CRGB ledsB[NUM_LEDS_B];
CRGB ledsC[NUM_LEDS_C];
CRGB ledsD[NUM_LEDS_D];
void setup() {
// Define the speed of the serial port
Serial.begin(115200);
}
void loop() {
// Set some counter / temporary storage variables
/// int cnt;
int cnt_A;
int cnt_B;
int cnt_C;
int cnt_D;
unsigned int num_leds; ///Total leds named in the HEADER(vixen software)
unsigned int d1, d2, d3;
int NLA;
int NLB;
int NLC;
int NLD;
// Begin an endless loop to receive and process serial data
for(;
// Set a counter to 0. This couter keeps track of the pixel colors received.
/// cnt = 0;
cnt_A = 0;
cnt_B = 0;
cnt_C = 0;
cnt_D = 0;
NLA=NUM_LEDS_A;
NLB=NUM_LEDS_B;
NLC=NUM_LEDS_C;
NLD=NUM_LEDS_D;
//Begin waiting for the header to be received on the serial bus
//1st character
while(!Serial.available());
if(Serial.read() != '>') {
continue;
}
//second character
while(!Serial.available());
if(Serial.read() != '>') {
continue;
}
//get the first digit from the serial bus for the number of pixels to be used
while(!Serial.available());
d1 = Serial.read();
//get the second digit from the serial bus for the number of pixels to be used
while(!Serial.available());
d2 = Serial.read();
//get the third digit from the serial bus for the number of pixels to be used
while(!Serial.available());
d3 = Serial.read();
//get the end of the header
while(!Serial.available());
if(Serial.read() != '<') {
continue;
}
while(!Serial.available());
if(Serial.read() != '<') {
continue;
}
// calculate the number of pixels based on the characters provided in the header digits
num_leds = (d1-'0')*100+(d2-'0')*10+(d3-'0');
// ensure the number of pixels does not exceed the number allowed
if(num_leds > NUM_LEDS) {
continue;
}
// Let the FastLED library know how many pixels we will be addressing
FastLED.addLeds<WS2811, DATA_PIN_A, GRB>(ledsA, NUM_LEDS_A);
FastLED.addLeds<WS2811, DATA_PIN_B, RGB>(ledsB, NUM_LEDS_B);
FastLED.addLeds<WS2811, DATA_PIN_C, BGR>(ledsC, NUM_LEDS_C);
FastLED.addLeds<WS2811, DATA_PIN_D, RGB>(ledsD, NUM_LEDS_D);
// Loop through each of the pixels and read the values for each color
do {
while(!Serial.available());
ledsA[cnt_A].r = Serial.read();
while(!Serial.available());
ledsA[cnt_A].g = Serial.read();
while(!Serial.available());
ledsA[cnt_A++].b = Serial.read();
--num_leds;
}
while(--NLA);
/////// Loop through each of the pixels and read the values for each color
do {
while(!Serial.available());
ledsB[cnt_B].r = Serial.read();
while(!Serial.available());
ledsB[cnt_B].g = Serial.read();
while(!Serial.available());
ledsB[cnt_B++].b = Serial.read();
--num_leds;
}
while(--NLB);
////// Loop through each of the pixels and read the values for each color
do {
while(!Serial.available());
ledsC[cnt_C].r = Serial.read();
while(!Serial.available());
ledsC[cnt_C].g = Serial.read();
while(!Serial.available());
ledsC[cnt_C++].b = Serial.read();
--num_leds;
}
while(--NLC);
// Loop through each of the pixels and read the values for each color
do {
while(!Serial.available());
ledsD[cnt_D].r = Serial.read();
while(!Serial.available());
ledsD[cnt_D].g = Serial.read();
while(!Serial.available());
ledsD[cnt_D++].b = Serial.read();
--num_leds;
}
while(--NLD);
// Tell the FastLED Library it is time to update the strip of pixels
FastLED.show();
// WOO HOO... We are all done and are ready to start over again!
}
}
Question 2: Since I am likely going to be using RS-485, is there anything i need to change with the code above to incorporate the data transmission? Or is it as simple as wiring up the receiving and transmitting ends of the modules themselves? Or does anyone know how I can set up the esp8266 for testing with this code when I get them in the mail?
Question 3: Knowing the goal listed at the top of this post, I want to use 2 teensy, probably 4-6 Nanos(for windows) and a mega for the solid-state relays, If I am using rs-485... that's a lot of serial ports, I will obviously need to get a USB hub extender. But at that expense, could I expect slower display speeds because of the large mess of data my computer will be spitting out from vixen? Would the esp8266 be better after all in that sense?